MongoDB’s document model is a revolutionary approach to database design that enables developers to store and query data with high efficiency and flexibility. Unlike traditional table-based relational databases, MongoDB utilises a document-oriented database structure that stores information in JSON-like documents with dynamic schemas. This offers a more natural way to represent data, as the document model can easily accommodate the complexity of real-world entities with nested structures and arrays, without requiring the rigid schema constraints of relational databases.
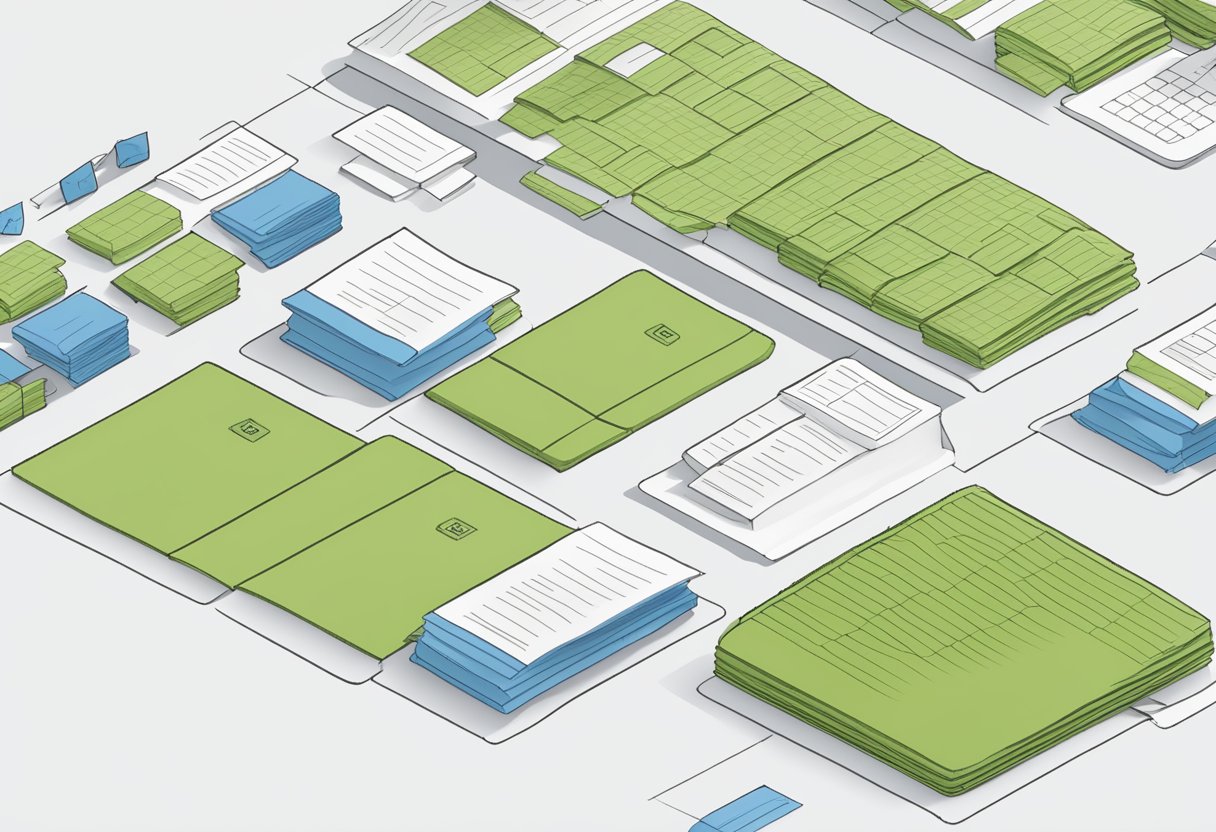
The document-centric model of MongoDB allows for the embedding of related information in a single document, which can lead to significant improvements in read performance and developer productivity. By storing related data together, MongoDB facilitates intuitive data retrieval and manipulation, making it a preferred choice for applications that demand agile data handling and swift development cycles. As the volume of data and demand for more responsive applications grow, the adaptability and scalability offered by MongoDB’s document model become increasingly paramount.
Key Takeaways
- MongoDB’s document model enhances data representation and query flexibility.
- Performance and development agility are improved by embedding related data.
- The document model supports scalable and adaptable database solutions.
Overview of MongoDB
MongoDB is a modern, NoSQL database that provides high performance, high availability, and easy scalability. Unlike traditional relational databases, MongoDB utilises a document model that is geared towards flexibility and ease of use. As you delve into MongoDB, you will discover that its basic unit of storage is a JSON-like document. This structure allows you to create complex hierarchies and store arrays and subdocuments without needing to predefine a schema.
In terms of data organisation, your data is grouped into collections, which are analogous to tables in a relational database. However, collections in MongoDB can hold diverse documents. For instance, one document in a collection could have different fields from another document. The documents are comprised of key-value pairs, which provide a dynamic schema that can evolve with your application’s needs.
Furthermore, MongoDB is designed to scale horizontally, using a process called sharding, which distributes the data across multiple machines. This approach provides you with a way to manage large data sets and high throughput operations effectively.
When interacting with your data, MongoDB supports a range of query mechanisms, including simple searches to more complex operations like text search and geospatial queries. These capabilities ensure that you are equipped to manage and retrieve your data efficiently in a variety of contexts.
Remember to explore the lessons provided by MongoDB University to deepen your understanding of MongoDB’s features and begin improving your database management skills.
Fundamentals of Document-Oriented Databases
In understanding MongoDB’s Document Model, you need to grasp the core principles of document-oriented databases, which are structured to handle data flexibly and intuitively.
Documents vs Traditional Relational Tables
Documents are the primary unit of data in MongoDB, contrasting sharply with the rows and columns of traditional relational tables. In a document database, each document stores related information together and can contain nested structures like lists and subdocuments. This is akin to how your real-world documents encompass a variety of information that doesn’t conform to the rigid structure of a table. Documents align more naturally with object-oriented programming, simplifying data manipulation in your code.
For instance, considering a user profile, a document in MongoDB would encapsulate all of the user’s details:
{
"username": "exampleUser",
"profile": {
"firstName": "John",
"lastName": "Doe",
"age": 30
},
"interests": ["coding", "cycling"]
}
In contrast, a traditional relational database would require multiple tables, one for user credentials, another for profiles, and a third to manage interests. To construct a full profile, a join operation is necessary, which can increase complexity and affect performance as data volume grows.
BSON Format: A Closer Look
MongoDB leverages BSON (Binary JSON) to store documents. BSON extends the popular JSON format to provide additional data types such as date and binary, which are not directly supported in standard JSON. Consider BSON as a bridge between the ease of JSON and the need for richer data types demanded by serious applications.
For example:
{
"item": "canvas",
"qty": 100,
"size": { "h": 28, "w": 35.5, "uom": "cm" },
"status": "A",
"lastModified": { "$date": "2023-01-08T08:00:00Z" }
}
Here, "lastModified"
includes a BSON date type, which JSON alone could not represent. This is invaluable as it allows for precise date and time handling within your database directly, thereby improving data consistency and easing interactions from your applications.
Designing a MongoDB Schema
When you’re constructing a MongoDB schema, two primary design patterns to consider are embedded documents and references and normalisation. Your choice will significantly impact performance and data consistency.
Embedded Documents Strategy
Utilising embedded documents allows you to store related data within a single document. This is ideal for data with a one-to-one relationship or for one-to-many relationships where you can predict the array size.
- Pros:
- Faster reads because required data is in the same document
- Avoids expensive join operations
- Cons:
- Document size limit (currently 16 MB)
- Can lead to data duplication and increased storage use
For instance, if you’re managing a user’s data with their address, an embedded model could be the most efficient strategy.
References and Normalisation
The approach of references and normalisation is more aligned with traditional relational databases, where you link documents using references.
- Pros:
- Flexibility in document structure
- Easier to update referenced data
- Cons:
- Requires additional queries to resolve references
- Potentially slower reads due to joins
An example would be an e-commerce platform where a product catalogue changes frequently, making referencing a better choice.
Querying and Indexing
In MongoDB, querying operates on the principle of leveraging the document model’s flexibility. When you need to retrieve data, your queries can specify a vast array of conditions and criteria. For example, if you require documents that match certain fields, you may construct a query including those field-value pairs. The document-oriented structure facilitates complex queries, allowing you to use operators like $and
, $or
, and $not
to refine your search further.
Indexing is crucial in enhancing the performance of such queries. By setting up indexes on document fields, you provide MongoDB with a structured pathway to efficiently locate data. This mechanism is particularly beneficial when dealing with large datasets. An effective index reduces the number of documents MongoDB must scan to find the relevant matches.
Here’s a basic explanation of index types:
- Single Field: Targets one field within your documents.
- Compound Index: Combines multiple fields for a single index.
- Multikey Index: Useful for indexing array fields, creating an entry for each element.
Indexes are necessary for optimal query execution—without them, MongoDB scans each document in a collection, which can lead to slower response times.
To manage your databases, collections, and documents more effectively, it’s beneficial to understand Atlas Data Explorer, which provides a user-friendly interface for querying and indexing operations.
In summary, adeptly using querying and indexing in MongoDB ensures efficient data retrieval and enhances the performance and scalability of your database operations.
Understanding MongoDB’s CRUD Operations
MongoDB organises data in flexible, JSON-like documents, enabling your applications to have data structures that can be modified over time. This flexibility facilitates the four main operations you can perform on data: create, read, update, and delete (CRUD).
Create Operations
When you create documents in MongoDB, you’re essentially adding new data into the database. Use the insertOne()
method if you’re adding a single record, or insertMany()
for inserting multiple records simultaneously. It’s vital to ensure your data adheres to any specified schemas or validation rules.
Read Operations
Reading documents is about querying the database to retrieve data. You can perform simple queries for specific documents using find()
or obtain a single document with findOne()
. Complex queries might involve sorting, filtering, and paginating your results to find exactly what you need.
Update Operations
To update documents, MongoDB offers methods like updateOne()
, updateMany()
, or findOneAndUpdate()
. These allow you to modify existing records in various ways, from changing a single value to more complex operations using update operators or array modifiers.
Delete Operations
Deleting documents is a critical operation that should be done with caution. The deleteOne()
and deleteMany()
methods enable you to remove documents from your collection. It’s crucial to target the right records, as deleted data cannot be easily restored without a backup.
Using these CRUD operations efficiently enables you to manage your MongoDB databases effectively, ensuring data integrity and optimal performance.
Scaling MongoDB
As your application grows, MongoDB provides robust solutions for scaling. You need to consider two main strategies: sharding and replication, each serving different scaling needs.
Sharding
Sharding in MongoDB is a method for distributing data across multiple machines. It involves splitting large datasets (collections) into smaller, more manageable pieces, called shards. Each shard is held on a different server, effectively spreading the load. This is particularly useful when your data size exceeds the capacity of a single machine. MongoDB uses a process called sharding
to ensure your queries are routed to the correct shard, thus maintaining performance even with large amounts of data.
Replication
Replication involves creating multiple copies of your data and storing them on different database servers. This process enhances data availability and resilience. In MongoDB, a group of servers that maintain the same data set are called a replica set. Each replica set has a primary node that handles all write operations and multiple secondary nodes that replicate the primary’s data. If the primary server fails, one of the secondary servers can automatically take over, minimizing downtime and preventing data loss. Replication is a key part of MongoDB’s strategy for ensuring high availability and data redundancy.
Data Modelling Best Practices
When approaching data modelling in MongoDB, it is crucial to align your data model with your application’s requirements. Considering the nature of document-oriented databases, the following best practices can enhance performance and scalability:
- Embedding vs. Referencing: Choose to embed related data in a single document for improved read performance, but only if the data is accessed together. Use referencing if the data grows unboundedly or is only occasionally accessed together. The Data Model Examples and Patterns documentation provides insight into these choices.
- Define Your Document Structure: Prioritise intuitive structuring of your documents by grouping related fields together. Remember, documents should correspond to objects in your code, which can help maintain a clear and manageable schema.
- Indexing: Efficient indexing is vital; create indexes that support your application’s query patterns. Avoid over-indexing as it can lead to unnecessary performance overheads.
- Field Names Consideration: Since field names are stored with every document, choose concise field names to reduce storage space.
- Balancing Duplication and Normalisation: Consider duplicating data where read performance is crucial, and normalisation where data integrity is key. Non-normalised data models can be better for read-heavy applications, while a more normalised form might suit applications where data integrity is paramount. An understanding of normalized versus non-normalized data models can be found detailed in an Amazon blog post.
- Use of Arrays: Only use arrays for fields that have a small number of elements as large arrays can degrade performance and complicate updates.
- Awareness of Document Growth: Limit updates that increase the size of a document to avoid moving documents on the disk, which can be performance impacting.
Performance Optimisation
In MongoDB, your database performance hinges on two pivotal aspects: how efficiently you can retrieve data, and how well you can process it. The adequacy of your indexing strategies and the optimisation of your aggregation pipelines are vital to these ends.
Indexing Strategies
When you establish indexes in MongoDB, you’re essentially creating specific paths to your data, which can dramatically speed up query performance. It is critical to understand your application’s query patterns to design effective indexes. For numerous queries involving a particular field, an index on that field can lead to substantial performance gains.
- Single-field Indexes: Start with indexing individual fields that are frequently queried.
- Compound Indexes: Create indexes on multiple fields when queries span several fields.
- Multikey Indexes: Use these for indexing array fields to enhance search within arrays.
By selecting the appropriate indexes, you prevent exhaustive collection scans, reduce I/O operations, and improve overall execution time of queries.
Aggregation Pipelines
The aggregation pipeline is a framework for data aggregation modelled on the concept of data processing pipelines. Your documents pass through multiple stages, each transforming the data step by step. Optimising these pipelines is crucial for the performance of complex data aggregation tasks in MongoDB.
- Project Early: Use
$project
to limit the fields early in your pipeline to reduce the amount of data processed. - Filter Early: Implement
$match
as early as possible to filter out unnecessary documents. - Use Indexes: Ensure stages use indexed fields to allow MongoDB to use index scans.
Clever structuring of your aggregation pipelines can lead to significant performance improvements, sometimes resulting in orders of magnitude faster operations.
Security Considerations
When managing your data with MongoDB’s document model, it’s crucial to prioritise security to protect sensitive information. Here are essential practices to consider:
- Access Control: Ensure that you implement robust authentication by enabling MongoDB’s built-in support for user authentication. Create user accounts with the least privileges necessary, thereby following the principle of least privilege.
- Network Security: Limit network exposure of your MongoDB server. Use firewalls or Virtual Private Networks (VPNs) to restrict which clients can connect to the database.
- Encryption: Protect data at rest by using Transparent Data Encryption, which encrypts the storage engine’s files. Additionally, enable TLS/SSL encryption for all clients and server communication to safeguard data in transit.
- Auditing: Track access and changes to the database by enabling MongoDB’s auditing capabilities to keep a record of activities.
- Regular Updates: Keep your MongoDB server updated. Apply patches and version upgrades to remedy known vulnerabilities.
- Backup and Recovery: Implement a backup strategy to ensure you can restore your data in case of a breach or data loss.
Remember, no single feature can secure your database completely; a layered approach to security is recommended. Always refer to official MongoDB security best practices for comprehensive guidelines.
For educational resources on how to secure MongoDB, consider enrolling in courses offered by MongoDB University.
Backup and Recovery Strategies
When managing your MongoDB database, it is essential to implement robust backup and recovery strategies to safeguard your data. There are several recognised methods for backing up MongoDB data:
- Full Backup: You create a complete copy of your database at regular intervals. This method ensures that you have all the data, but may require more storage space and time.
- Incremental Backup: With this strategy, you only backup data that has changed since your last backup. It’s more storage-efficient and quicker but requires a full backup for the initial setup.
- Differential Backup: This technique involves backing up only the changes made since the last full backup. It strikes a balance between the previous two methods.
Here are the steps you can take to ensure effective backup and recovery:
- Regularly Schedule Backups: Determine the frequency of your backups based on your data volatility and business requirements.
- Test Your Recovery Plan: Periodically testing the restoration process is critical to ensure that your strategy is effective.
- Utilise MongoDB Tools: Tools like
mongodump
andmongorestore
facilitate the backup and restoration process.
For more detailed guidance, reference resources like the free course on MongoDB Atlas Backup & Recovery or explore thorough recommendations on backup methods in An Introduction To Backup And Restore.
Remember, having a consistent and testable backup plan in place is crucial. It can be the difference between a minor inconvenience and a major business crisis.
Frequently Asked Questions
In this section, you’ll find answers to common questions about MongoDB’s document model, enabling you to better understand its structure and functionality.
How does document structure function within MongoDB?
Within MongoDB, the document structure functions as the basic unit of data, analogous to a row in a relational database. These documents are stored in a JSON-like format called BSON, which allows for a more flexible schema and the storage of complex hierarchies.
Can you demonstrate an example of a MongoDB data model?
An example of a MongoDB data model might include a ‘Users’ collection where each document represents a user with fields for name, email, and an embedded array of ‘Posts’ that the user has created, illustrating an embedded one-to-many relationship within the same document.
In what way do MongoDB schemas differ from traditional relational database schemas?
MongoDB schemas are not enforced by default, meaning they are flexible and allow for varying fields across documents. This contrasts with traditional relational database schemas that require rigid, predefined structures and relations between tables.
Could you provide a detailed explanation of document databases with examples?
Document databases like MongoDB store data in documents rather than tables or rows, allowing for nested data structures and flexible schemas. For example, a blog post document might contain comments within it as an embedded array, eliminating the need for a separate comments table.
What are the distinctions between a model and a schema in the context of MongoDB?
In MongoDB, a model refers to the compiled version of the schema and acts as a constructor for creating documents. The schema itself is a structure that defines the shape and contents of documents within a collection, dictating the types of data and their relationships.
How does MongoDB manage the relationship between documents and collections?
MongoDB manages relationships by embedding related data within documents, reducing the need for separate read operations, or by using references where one document contains a reference to another through an object ID, similar to foreign keys in relational databases but without enforcing constraints.